The personal view on the IT world of Johan Louwers, specially focusing on Oracle technology, Linux and UNIX technology, programming languages and all kinds of nice and cool things happening in the IT world.
Monday, May 30, 2011
Solved: REST is not enabled
Reason for this is that you started the database without the --rest option. To do this you can start the database from your MongoDB bin directory like "./mongod --rest". This will solve the issue. It is however not explaining what --rest is doing.
According to Wikipedia REST is nothing more than Representational State Transfer (REST) is a style of software architecture for distributed hypermedia systems such as the World Wide Web. The term Representational State Transfer was introduced and defined in 2000 by Roy Fielding in his doctoral dissertation.Fielding is one of the principal authors of the Hypertext Transfer Protocol (HTTP) specification versions 1.0 and 1.1. Conforming to the REST constraints is referred to as being ‘RESTful’.
When adding the --rest when starting MongoDB will enable you to do a lot more and interact in more ways to your MongoDB database from your webinterface. This however means that also other people can connect and do more than without the --rest option. For this reason it is good to take into consideration if you want the --rest option activated and if so how you want to secure access to it. You can for example think about having a firewall rule (local or in your network) which states that only certain machines can access the web interface port.
Render XML into DIV using AJAX and XSL
For this you will need a small java script. I build the below example. This example is showing how you can load dynamically a XML file in combination with a XSL file, have it transformed into HTML and display it in a DIV element in your page. The nice thing about this approach is that you only have to create your XML file and have the rendering done on your clients workstation. If you update the content of the XML your page will automatically contain new information the next time a user requests the page. A second benefit is that if you want to change the layout of your page you have to only update you XSL style sheet. The example I provide below is quite simple where I use AJAX in combination with XML and XSL to dynamically rendered HTML content of a CD catalog on a page when clicked on a link. The example is not really useful as a page/application however it is showing how you can use the script.
The example contains 2 XML files, 1 XSL file and a HTML file. If you place them all in the same location and open test.html you will see how it is working.
test.html
<html>
<head>
<script>
function loadXMLDoc(dname) {
if (window.XMLHttpRequest) {
xhttp = new XMLHttpRequest();
} else {
xhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
xhttp.open("GET", dname, false);
xhttp.send("");
return xhttp.responseXML;
}
function displayResult(source,styledoc) {
xml = loadXMLDoc(source);
xsl = loadXMLDoc(styledoc);
//set a   to make prevent FF to append the text by double click
document.getElementById("mydiv").innerHTML = " ";
// code for IE
if (window.ActiveXObject) {
ex = xml.transformNode(xsl);
document.getElementById("mydiv").innerHTML = ex;
}
// code for Mozilla, Firefox, Opera, etc.
else if (document.implementation && document.implementation.createDocument) {
xsltProcessor = new XSLTProcessor();
xsltProcessor.importStylesheet(xsl);
resultDocument = xsltProcessor.transformToFragment(xml, document);
document.getElementById("mydiv").appendChild(resultDocument);
}
}
</script>
</head>
<body>
<a onclick="displayResult('cdcatalog.xml','cdcatalog.xsl')" href="javascript:void(0);">catalog 1</a>
<a onclick="displayResult('cdcatalog2.xml','cdcatalog.xsl')" href="javascript:void(0);">catalog 2</a>
<div id="mydiv">
</div>
</body>
</html>
cdcatalog.xml
<?xml version="1.0" encoding="ISO-8859-1"?>
<catalog>
<cd>
<title>Empire Burlesque</title>
<artist>Bob Di.</artist>
<country>USA</country>
<company>Columbia</company>
<price>10.90</price>
<year>1985</year>
</cd>
</catalog>
cdcatalog2.xml
<?xml version="1.0" encoding="ISO-8859-1"?>
<catalog>
<cd>
<title>Some title </title>
<artist>Some artist</artist>
<country>USA</country>
<company>Columbia</company>
<price>10.90</price>
<year>1985</year>
</cd>
<cd>
<title>Some othertitle </title>
<artist>The artist</artist>
<country>USA</country>
<company>Columbia</company>
<price>11.40</price>
<year>1985</year>
</cd>
</catalog>
cdcatalog.xsl
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<html>
<body>
<h2>My CD Collection</h2>
<table border="1">
<tr bgcolor="#9acd32">
<th align="left">Title</th>
<th align="left">Artist</th>
</tr>
<xsl:for-each select="catalog/cd">
<tr>
<td><xsl:value-of select="title" /></td>
<td><xsl:value-of select="artist" /></td>
</tr>
</xsl:for-each>
</table>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
Friday, May 27, 2011
Running MongoDB on a mac
I a couple of simple steps you can get a working copy of MongoDB on your local mac workstation. If you follow these steps you will be set to start exploring MongoDB
1) Go to the mongodb.org website and download the needed .tgz file.
2) Unpack the file and copy the files to a location where you want to have your MongoDB binary files.
3) Create a directory /data/db this is the default location where your data will be stored, you have to create this manually.
4) Go to your MongoDB bin directory and start mongod
5) Open a second terminal and execute in the MongoDB bin directory the mongo command.
Step 5 will put you in the MongoDB shell and you are ready to go and explore the options of MongoDB. This is all you need to start exploring MongoDB.
As a example below you can see the output of the mongod command on my local workstation:
Johan-Louwerss-MacBook-Air:bin suntac$ ./mongod
./mongod --help for help and startup options
Fri May 27 14:48:50 [initandlisten] MongoDB starting : pid=17438 port=27017 dbpath=/data/db/ 32-bit
** NOTE: when using MongoDB 32 bit, you are limited to about 2 gigabytes of data
** see http://blog.mongodb.org/post/137788967/32-bit-limitations
** with --dur, the limit is lower
Fri May 27 14:48:50 [initandlisten] db version v1.8.1, pdfile version 4.5
Fri May 27 14:48:50 [initandlisten] git version: a429cd4f535b2499cc4130b06ff7c26f41c00f04
Fri May 27 14:48:50 [initandlisten] build sys info: Darwin broadway.local 9.8.0 Darwin Kernel Version 9.8.0: Wed Jul 15 16:55:01 PDT 2009; root:xnu-1228.15.4~1/RELEASE_I386 i386 BOOST_LIB_VERSION=1_40
Fri May 27 14:48:50 [initandlisten] waiting for connections on port 27017
Fri May 27 14:48:50 [websvr] web admin interface listening on port 28017
Wednesday, May 25, 2011
MongoDB with BJSON, How could I have missed it
Every now and then you have the feeling "how could I have missed this". In my case this came today when I was looking for a quick to deploy and easy to use database platform. I am currently working on a little project to automate some of my tasks at home and for this I am in need to store information. One options was storing it in XML files on the filesystem. However, due to the fact I am a Oracle guy I tend to look for a database solution first. Having a Oracle database running for the storing of this information is a little overkill and I wanted it to be standalone so using one of my already running Oracle databases for this was not an option.
MySql, PostgreSQL and some other things came to mind however when doing some fun google work I came to a database type I did never heard of before and looking at it the phrase "how could I have missed this" came to mind.
MongoDB is a easy to deploy database and has some great options. Maybe not for this little project but for a lot of other projects this can come in handy. MongoDB is not having schema's such as most databases and documents are stored as BJSON (Binary JavaScript Object Notation)
If you look at he way how to interact and query a MongoDB it somehow comes very naturally if you play with it. Below you can find some information from youtube from a Google User Group meeting where Will Shulman, founder and CEO of MongoLabs, give us an excellent overview on how to persist our mobile app data in the cloud using the super-scalable and amazingly developer-friendly MongoDB back-end.
Part 1:
Part 2:
Posted via Johan Louwers his Posterous page to his blogger page.
Solved: android tab layout example
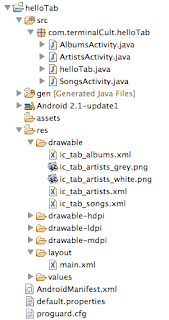
I have been completing the "Tab Layout" example and ran into some error's and found that more people are having difficulty completing the "Tab Layout" example. Reason for this is that the google example is a example and not a step by step guide on how to program for Android. As I found some well discussed issues with this example I found that a lot of people have difficulties with this example.
To help those people you can find the source of the example I build. The main issue I personally encountered in this example is the content of the androidmanifest.xml file. I hope this post will give you some more insight in how to develop a tab layout and will help you understand how to work with the example given by Google.
AlbumsActivity.java
package com.terminalCult.helloTab;
//import some stuff we need to get the example code running
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
// starting the class
public class AlbumsActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textview = new TextView(this);
//show some text to state which tab this is
textview.setText("This is the albums tab");
setContentView(textview);
}
}
ArtistsActivity.java
package com.terminalCult.helloTab;
//import some stuff we need to get the example code running
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
// starting the class
public class ArtistsActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textview = new TextView(this);
//show some text to state which tab this is
textview.setText("This is the Artists tab");
setContentView(textview);
}
}
SongsActivity.java
package com.terminalCult.helloTab;
//import some stuff we need to get the example code running
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
// starting the class
public class SongsActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textview = new TextView(this);
//show some text to state which tab this is
textview.setText("This is the songs tab");
setContentView(textview);
}
}
helloTab.java
package com.terminalCult.helloTab;
import android.os.Bundle;
import android.app.TabActivity;
import android.content.*;
import android.content.res.*;
import android.widget.*;
public class helloTab extends TabActivity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Resources res = getResources(); // Resource object to get Drawables
TabHost tabHost = getTabHost(); // The activity TabHost
TabHost.TabSpec spec; // Resusable TabSpec for each tab
Intent intent; // Reusable Intent for each tab
// Create an Intent to launch an Activity for the tab (to be reused)
intent = new Intent().setClass(this, ArtistsActivity.class);
// Initialize a TabSpec for each tab and add it to the TabHost
spec = tabHost.newTabSpec("artists").setIndicator("Artists",
res.getDrawable(R.drawable.ic_tab_artists))
.setContent(intent);
tabHost.addTab(spec);
// Do the same for the other tabs
intent = new Intent().setClass(this, AlbumsActivity.class);
spec = tabHost.newTabSpec("albums").setIndicator("Albums",
res.getDrawable(R.drawable.ic_tab_albums))
.setContent(intent);
tabHost.addTab(spec);
intent = new Intent().setClass(this, SongsActivity.class);
spec = tabHost.newTabSpec("songs").setIndicator("Songs",
res.getDrawable(R.drawable.ic_tab_songs))
.setContent(intent);
tabHost.addTab(spec);
tabHost.setCurrentTab(2);
}
}
ic_tab_albums.xml
ic_tab_artists.xml
ic_tab_songs.xml
main.xml
AndroidManifest.xml
Monday, May 23, 2011
Solved; Android Emulator not starting
When you start working with the Android development tools to develop your first Android Application you will, most likely, be using the Eclipse tools provided and you will most likely start with a HelloWorld example. HelloWorld examples are used in almost every programming guide to show you a "Hello World" text. It is considered the most simple application of showing something on the screen. Google has also provided a Hello World example which will show you how to work with eclipse plugin and how the emulator is working. If you are following the steps in the development guide from Oracle you have to run your application at some point. This is however a point I feel a lot of people will drop it if they are not really determine. The reason is not that it is complex, the reason is that you have to wait and you are not warn that you have to wait.
When you run a example the first time you expect it to be on the screen directly. However when you run your HelloWorld example you see the Android Emulator coming up with on the word "ANDROID" in a black screen and not the expected "Hello World" text. Reason for this is quite simple as is the solution. When you click run for the first time the entire emulator has to be started and the "ANDROID" message is the first stage of the boot screen. Solution; wait. After some time you will see the android splash screen coming up and short after that your application. Now you also have a complete Android device and the basic Android OS you can use and play with. Meaning, if you only see "ANDROID" in your screen when you run your application for the first time, do not be afraid, you most likely did not make a mistake you simply have to wait for one or two minutes for the emulator to boot completely. After that you can go back to your development environment and change whatever you like. If you click run the second time and you have closed down your emulator only the new code will be loaded into the emulator and it will not have to be rebooted.Posted via Johan Louwers his Posterous page to his blogger page.
Oracle Complete Positioning Versus Competetion
During the "Oracle Day 2011" organized by the Oracle User Group in Estonia OUGE a talk was given by Shahin Taromsari on the subject "Complete Positioning Versus Competetion". Shahin is given a very nice view on the Oracle strategy for the upcoming time in a relaxed way and providing a insight in what it will mean in the upcoming time for your company or what it can mean in the upcoming time. After the merger with Sun Microsystems they have now the option to build the entire stack in one company. They can build the hardware and the software which enables Oracle to create the best solutions in the market. Even do they are able to do so, in my opinion, Oracle is still a software vendor playing to be a hardware vendor. They have a hard time to make the shift to also think like hardware vendors think. This process will take some time however I do have a feeling that in the end they will succeed. A long way however has to be taken before it will become the success that it could be. I already understand from a lot of people that some frustration is raising within the relationship with the new Sun Microsystems part of the company and clients as former Sun employees now have to confirm to the way of working as set by Oracle. This way of working is a software vendor way of working and not a hardware vendor way of working.
I am confident that Oracle is having a lot of smart people working on this culture issue and will come with a solution, I only hope this will come quick and that they understand that change is needed on both the software side as the hardware side. People of research and development departments tend to work already together in a great way and started engineering new products that work together in a better way now the rest of the company will have to follow.
Posted via Johan Louwers his Posterous page to his blogger page.
Thursday, May 19, 2011
Installing a Android dev environment
Downloading the SDK can be done from the google android developers page. Just download the zip file and unpack it somewhere on your machine where you think it is appropriate to store it.
The second part is to install the eclipse plugin. For some reason it is also possible to download all the parts of the SDK from the eclipse plugin option. In my case it was even needed as for some reason I was unable to associate the downloaded SDK with the eclipse plugin.
To install the eclipse plugin you go to "help" -> "Install new software". here you can add the location where Google is storing the eclipse plugin; https://dl-ssl.google.com/android/eclipse/
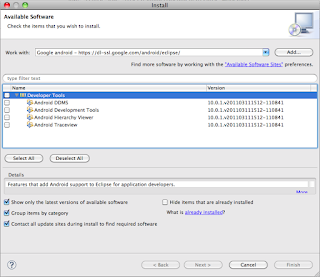
In my case I had to look under "installed Packages" and do a "update all" for some reason as it was unable to associate the downloaded SDK with the eclipse plugin.
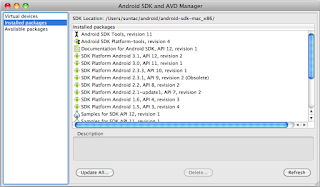
Wednesday, May 18, 2011
Using Oracle Business Process Management
I was recently asked to help some people with looking into the processes and procedures of one of our clients at Capgemini. I was involved at this customer due to the fact that they have a large Oracle landscape which is used across the EMEA region and as I am already familiar with the customer and I have done already work in setting up processes and procedures to connect demand organizations within the Capgemini rightshore model I started looking into the situation. The question was how can we advise the customer to change some of the processes and procedures concerning Incident management so the service towards the end users is faster and more cost effective.
After some time of whiteboard sessions a couple of models came to my mind. The difficulty however with those kind of models is to find out which one will work best in a realtime situation and where will we find bottlenecks. I installed the Oracle BPM part of Oracle jDeveloper a couple of months ago however did not had the option to play with it. It turned out that we where able to create all the scenario's within the BPM tool and simulate all kind of situations. By using Oracle BPM I was able to quickly model the different scenarios and play situations on them. By doing so we could simulate a realtime situation and change the processes and procedures in our final advise so the customer can implement them.If you are ever in the need of simulating business processes you should give Oracle BPM a look. Below you will find a youtube movie and a presentation which give you some more info on the subject and will show how to model a test situation.The below video is made by Marcus Davies from Integrella which shows you quickly how you can model a simulation within Oracle jDeveloper.Posted via Johan Louwers his Posterous page to his blogger page.
Monday, May 16, 2011
Oracle Roadmap to Enterprise Cloud Computing
As some of you might know I have been busy developing the new Capgemini Cloud Computing strategy and solutions the past half year for Oracle products. Due to this I have been talking to Oracle a lot and we both Capgemini and Oracle have been working together the past half year to get things in both a business as a technology way up and running. We are at the doorstep of launching this new platform so from time to time I am already allowed to slip some information to the outside world.
It is interesting to watch that the combination Capgemini and Oracle have a strong research and development partnership during the build of our new cloud platform. It is also nice to see that Oracle is running their own roadmap which includes quite some of the same viewpoints as we are having within the team inside Capgemini. This is not very surprising as we are developing this together with Oracle. To me personally and to Capgemini it comes as a no-brainer that cloud computing is the future and the road we should take and which we are taking. However a lot of different opinions within do exist within the IT world on what cloud computing is, which way we should go and what the best way is to create a cloud computing platform. I am not diving into this discussion at this moment. Some very good mailing lists do exist on the future and the roadmap of cloud computing and if you are interested in discussing on the future of cloud computing I would invite you to join one of them.Within this post I would like to share a presentation from Oracle which is given by Rex Wang who is vice president and is sharing the Oracle roadmap and vision on cloud computing. A very interesting presentation to watch if you are interested in the cloud computing topic. This presentation is on enterprise cloud computing so it is not touching public cloud computing. In my personal opinion we will see a lot of things happening in the field of private and enterprise cloud computing as companies do tend to prefer having data inside their own network and are getting more and more data to process and the need for more and more on-demand computing power.Saturday, May 14, 2011
Oracle Advanced Compression
Oracle Advanced compression is a database option which from Oracle 11G finally getting to work in a more proper way than before. Oracle Advanced Compression can help you lower your storage costs by compressing the data in your database or as Oracle like to state it: "Advanced Compression, an option introduced in Oracle Database 11 g Enterprise Edition, offers a comprehensive set of compression capabilities to help organizations reduce costs, while maintaining or improving performance. It significantly reduces the storage footprint of databases through compression of structured data (numbers, characters) as well as unstructured data (documents, spreadsheets, XML and other files). It provides enhanced compression for database backups and also includes network compression capabilities for faster synchronization of standby databases."
Oracle advanced compression is a payed option in your database, for the latest pricing you can refer to the Oracle Global Pricelist which can be downloaded from the Oracle website. As this is a payed option you have to be sure that you will be able to get your return on investment before you begin. To be able to build a business case for purchasing Oracle Advanced Compression Oracle is providing us with some tools which you can run on your database to check the level of compression you can establish. The "compression advisor" which you can download at the Oracle website needs to be compiled into your database is providing you some basic information and still needs a lot of manual labor in running it on your entire database and after that some time needs to be spend on understanding and calculating the results before you can use them in your business case to validate your ROI of this database option.For a resent project at Capgemini I have been developing a more robust and more usable implementation of the Oracle Compression Advisor in combination with a default calculation to calculate your ROI and helping you to generate a business case. If you are interested in looking into the options of Oracle Advanced Compression in your database, please feel free to contact me. The current version of the "Improved Compression Advisor" is currently still in testing at the moment I write this document and needs some manual steps of moving the results into excel however this is about to change within the coming weeks and it will most likely be placed online under a GNU/GPL license model.
For more information on advanced compression a large amount of information can be found online in the form of Documentation from Oracle also some good video's from speeches are posted online; Christina Horsoe uploaded a video to vimeo with a presentation on a case study done by DSP. You can see the video below, the comment they provided to the video is the following. It is quite nice to have a quick look if you where not able to join the presentation yourself. "Our case study is what the customer has termed a “Next Generation” Billing Project at a leading European Telecoms company. The system was required to consolidate billing in the 13 different countries around the world and needed to be available 24/7. . The database is multi-terabyte containing billions of Call Data Records. The presentation will take a look at three significant challenges the project had to overcome and illustrate how that the Oracle Partitioning and the Oracle Advanced Compression Options’ was utilised to solve them. Oracle partitioning was used to partition the data simplifying the necessary archival process leading to £18,000 per month storage savings. Our performance challenge was met using partition pruning and also partition-wise joins, which led to performance improvements of over 100 times. Finally, Oracle Advanced Compression was tested and proved to achieve storage saving of 2.8 to 1 resulting in a £77,000 saving in the first year."OracleVideo has posted a short video on youtube which also is concerning Oracle Advanced Compression named "Storage Management with Oracle Database 11g"
If you are in need of more information or want to discuss more details and options on lowering your storage costs and implementing Oracle Advanced Compression please feel free to contact me via mail or send me a direct twitter message asking me to contact you.
Posted via email from Johan Louwers posterous page by Johan Louwers.
Google I/O 2011: Honeycomb Highlights
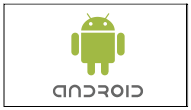
Android 3.1 which is the new version of android is bringing some very nice new features for developers. I recently purchased a Android tablet to play around with and looking into the development guides and the information provided by Google this can become a very interesting time. The Android 3.1 platform adds refinements and new capabilities that developers can build on, to create powerful and engaging application experiences on tablets and other large-screen devices.
Tuesday, May 10, 2011
Oracle Metalink security considerations
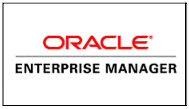
The below message is the message posted by Oracle on Metalink:
//--------------
Wikipedia defines social engineering as follows::
“Social engineering is the act of manipulating people into performing actions or divulging confidential information, rather than by breaking in or using technical cracking techniques. While similar to a confidence trick or simple fraud, the term typically applies to trickery or deception for the purpose of information gathering, fraud, or computer system access; in most cases the attacker never comes face-to-face with the victim. Social Engineering has also been employed by bill collectors, skiptracers, and bounty hunters.”
Oracle has become aware of an increasing number of attempts to get access to My Oracle Support by using social engineering techniques such as impersonating real users / employees of a company – but using a misspelled mail account domain (e.g., john.doe@oracle.com versus john.doe@0racle.com). By impersonating a real user, not only will the fraudulent user get unauthorized access to MOS, but would also be able to access the service requests of the targeted company, which may contain confidential information.
Because customer User Administrators are responsible for approving new users against their company CSI(s), they should be on alert for this growing threat and double-check all users they approve or have approved in the past. In addition, Oracle takes this threat very seriously and will disable any account / user that it identifies as impersonating someone else.
If – as a customer User Administrator – you identify but can’t disable a fraud user, please log a non-technical Service Request and Oracle Support will ensure proper actions are taken.
Thank you,
Your support team
//--------------
This brings me back on one of the topics I already have discussed internally a couple of times and with customers. What do you want to share with Oracle from a security point of view. Not only talking about what content do you discuss with a support engineer, also what do you have for example Oracle Enterprise Manager upload to Metalink. It is possible to connect Oracle Enterprise Manager to Metalink and have it share information about the systems you are running. This way it will show you information which it retrieves from Metalink on what patches might need to be applied and when you log a service request you can simply select the system you have a question about and the support engineer will have all the information at hand. In the below screenshot you can see how you can connect your Oracle Enterprise Manager. This is a screenshot of an older version, in the latest version some issues might arise. I have been looking into this together with Henk Nap who has posted some information on this on his weblog.
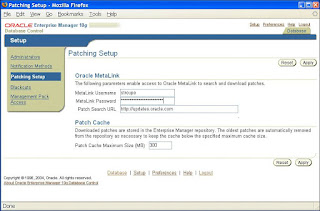
Friday, May 06, 2011
Oracle eBS internet Explorer certification Matrix.
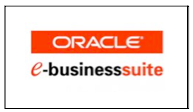
Sometimes I just have my blog to write reminders for myself (and for others) so they can quickly find some information on a specific topic. This is one of those posts. I am currently requested to look into the certification of systems used at a customer. One of the questions is if it is certified to upgrade Internet Explorer to version 9 in combination with Oracle e-Business Suite.
If you talk about Oracle e-Business Suite and browsers and java versions you tend to end up always on the weblog of Steven Chan. Also on the certification of Internet Explorer 9 for Oracle e-Business Suite steven has written a blogpost on his corporate Oracle blog. The latest (at this moment) post on this subject dates from 7-OCT-2010 and it states:
"IE 9 is available via a public platform preview today. We've been working with the latest IE9 beta, but it but hasn't been certified with the E-Business Suite yet."
As this is already a somewhat older blogpost I did a quick check with the people from Oracle and was directed to two metaling notes which provide a certification matrix for Oracle e-Business Suite.
Recommended Browsers for Oracle Applications 11i (Metalink Note 285218.1)
Recommended Browsers for Oracle Applications 12 (MetaLink Note 389422.1)
From the document you can cleary see that Oracle has NOT certified IE 9 at this moment. In the future please do refer to one of those two documents as they will be updated when things change.