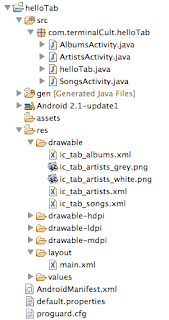
I have been completing the "Tab Layout" example and ran into some error's and found that more people are having difficulty completing the "Tab Layout" example. Reason for this is that the google example is a example and not a step by step guide on how to program for Android. As I found some well discussed issues with this example I found that a lot of people have difficulties with this example.
To help those people you can find the source of the example I build. The main issue I personally encountered in this example is the content of the androidmanifest.xml file. I hope this post will give you some more insight in how to develop a tab layout and will help you understand how to work with the example given by Google.
AlbumsActivity.java
package com.terminalCult.helloTab;
//import some stuff we need to get the example code running
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
// starting the class
public class AlbumsActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textview = new TextView(this);
//show some text to state which tab this is
textview.setText("This is the albums tab");
setContentView(textview);
}
}
ArtistsActivity.java
package com.terminalCult.helloTab;
//import some stuff we need to get the example code running
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
// starting the class
public class ArtistsActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textview = new TextView(this);
//show some text to state which tab this is
textview.setText("This is the Artists tab");
setContentView(textview);
}
}
SongsActivity.java
package com.terminalCult.helloTab;
//import some stuff we need to get the example code running
import android.app.Activity;
import android.os.Bundle;
import android.widget.TextView;
// starting the class
public class SongsActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textview = new TextView(this);
//show some text to state which tab this is
textview.setText("This is the songs tab");
setContentView(textview);
}
}
helloTab.java
package com.terminalCult.helloTab;
import android.os.Bundle;
import android.app.TabActivity;
import android.content.*;
import android.content.res.*;
import android.widget.*;
public class helloTab extends TabActivity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Resources res = getResources(); // Resource object to get Drawables
TabHost tabHost = getTabHost(); // The activity TabHost
TabHost.TabSpec spec; // Resusable TabSpec for each tab
Intent intent; // Reusable Intent for each tab
// Create an Intent to launch an Activity for the tab (to be reused)
intent = new Intent().setClass(this, ArtistsActivity.class);
// Initialize a TabSpec for each tab and add it to the TabHost
spec = tabHost.newTabSpec("artists").setIndicator("Artists",
res.getDrawable(R.drawable.ic_tab_artists))
.setContent(intent);
tabHost.addTab(spec);
// Do the same for the other tabs
intent = new Intent().setClass(this, AlbumsActivity.class);
spec = tabHost.newTabSpec("albums").setIndicator("Albums",
res.getDrawable(R.drawable.ic_tab_albums))
.setContent(intent);
tabHost.addTab(spec);
intent = new Intent().setClass(this, SongsActivity.class);
spec = tabHost.newTabSpec("songs").setIndicator("Songs",
res.getDrawable(R.drawable.ic_tab_songs))
.setContent(intent);
tabHost.addTab(spec);
tabHost.setCurrentTab(2);
}
}
ic_tab_albums.xml
ic_tab_artists.xml
ic_tab_songs.xml
main.xml
AndroidManifest.xml
3 comments:
Last few codes don't show up.
Ubuntu, Google Chrome
I cannot read the content of:
ic_tab_albums.xml
(blank line)
(blank line)
...
ic_tab_artists.xml
(blank line)
(blank line)
...
The same situation for all other .xml
The reason why it is not displayed is because the poster is posting XML in the web page inside of a pre tag. It is a webpage so the XML needs to be HTML encoded to show up correctly. However you can view the web pages source and find the xml inside the PRE tags, but it is a hassle.
I copied the content of the PRE tags to notepad++ and did a replace of br tags with \r\n. Then I could read it.
Post a Comment